Introduction
How do computers understand what we say or write? That’s where Natural Language Processing with Python shines! Natural Language Processing with Python is all about teaching machines to handle human language – think text or speech. You see Natural Language Processing with Python in chatbots, translation tools, and voice assistants like Alexa.
Why Python? Because Natural Language Processing with Python is made easy with libraries like NLTK, spaCy, and transformers. In this guide, we’ll dive deep into Natural Language Processing with Python, covering theory, code, and a handy PDF at the end!
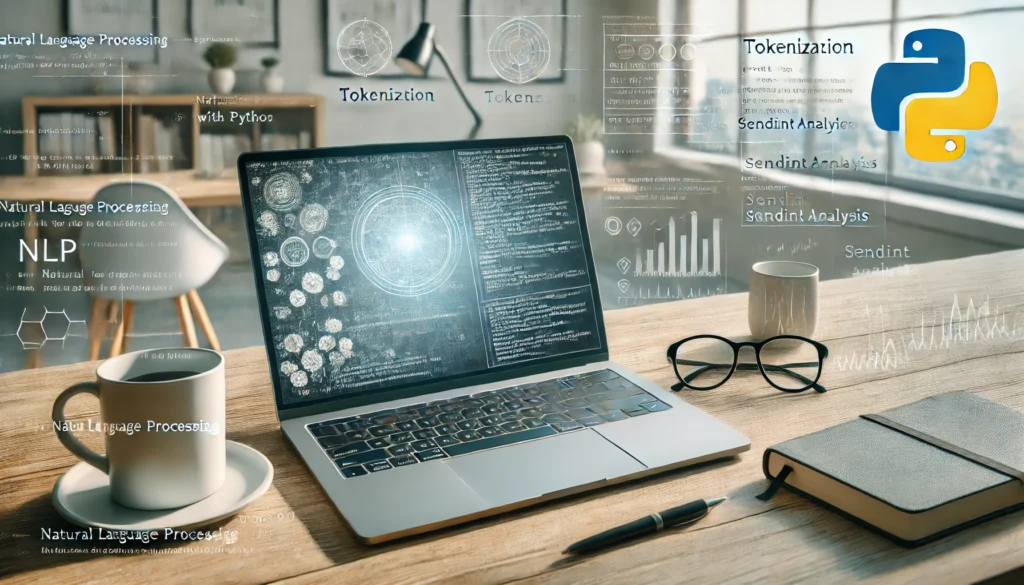
Importance of NLP
Helps in text classification (spam detection, fake news detection)
Enables sentiment analysis for customer reviews and social media monitoring
Enhances chatbots and virtual assistants
Facilitates machine translation (Google Translate)
Supports speech recognition applications like Siri and Alexa
Basics of NLP
Let’s start with the basics of NLP. Here’s what you need to know:
- Tokenization: In NLP, this splits text into words or “tokens”. Theory says it’s the first step to break down language for machines. Example: “I love coding” → [“I”, “love”, “coding”].
- Stop Words: NLP removes common words like “is” or “the” to focus on what matters. The idea is to clean up the text.
- Stemming/Lemmatization: With NLP, words like “running” turn into “run”. This groups similar words for better analysis.
These are the starting points of NLP
Setting Up Python for NLP
To begin Natural Language Processing with Python, set up your environment:
- Install Libraries: Run these commands:
pip install nltk
pip install spacy
import nltk
nltk.download('punkt') # For tokenization
print("Ready for NLP!")
Hands-On Coding: NLP Projects
Let’s get practical with Natural Language Processing with Python through some projects.
Step 1: Cleaning Text
Theory: Natural Language Processing with Python cleans text to remove noise and keep the good stuff.
Code:
import nltk
from nltk.tokenize import word_tokenize
text = "I am eating food and enjoying it"
words = word_tokenize(text)
print(words)
from nltk.corpus import stopwords
nltk.download('stopwords')
stop_words = set(stopwords.words('english'))
filtered_words = [word for word in words if word.lower() not in stop_words]
print(filtered_words)
Output: [‘eating’, ‘food’, ‘enjoying‘]
Step 2: Sentiment Analysis
Theory: Natural Language Processing with Python checks if text is positive or negative by scoring words.
Code:
from textblob import TextBlob
review = "This movie is awesome!"
blob = TextBlob(review)
sentiment = blob.sentiment.polarity
print(sentiment)
Output: 0.5 (Positive!)
Step 3: Named Entity Recognition (NER)
Theory: NLP tags names or places using context.
Code:
import spacy
nlp = spacy.load("en_core_web_sm")
text = "John lives in New York and was born on 15 March."
doc = nlp(text)
for ent in doc.ents:
print(ent.text, ent.label_)
John PERSON
New York GPE
15 March DATE
Advanced NLP
Here’s some advanced Natural Language Processing with Python:
- Word Embeddings: In NLP words become numbers based on meaning – “cat” and “kitten” are close.
- Transformers and BERT: These power NLP by understanding full sentences.
Code:
from transformers import pipeline
classifier = pipeline("sentiment-analysis")
result = classifier("I love Python!")
print(result)